Biased Typeahead¶
This section shows how typeahead results can be biased using a map. We focus on biasing the typeahead suggestions and not on typeahead or map rendering. To understand typeahead and how to set it up see previous section typeahead. To render a map we use the open source openlayers 3 mapping library, as shown in section :doc: ‘Render map <show_map>`.
Biasing suggestions¶
Biasing typeahead suggestions is easy using GeoIntelligence’s mapperjs typeahead library. The library is based on Twitter’s typeahead.js library and internally uses the Mapper Server Maps API for geocoding addresses.
The typeahead component has a mapLocation property that accepts an object with lat, lon properties. By setting this property you can bias the typeahead results:
typeahead.mapLocation = { lat: 37.9839375, lon: 23.7451027};
By setting mapLocation to null you are back to unbiased suggestions.
Example¶
In order to implement suggestions biasing you first have to capture the map location. You can do that with openlayers by listening to map events:
map.on('moveend', function (e) {
var latlon = ol.proj.toLonLat(map.getView().getCenter(), 'EPSG:3857');
typeahead.mapLocation = { lat: latlon[1], lon: latlon[0] };
});
The snipcode captures the center of the map and uses it to bias typeahead. However by implementing biasing this way the user will get biased results even if the map viewport contains the whole country. We can do better than that by biasing the results only if the user has zoomed enough.
The following code fragment on top of getting the map viewport center it also acquires the map zoom level. If the user has zoomed in enough then we bias the typeahead results, otherwise we can assume that he searches globally:
map.on('moveend', function (e) {
var latlon = ol.proj.toLonLat(map.getView().getCenter(), 'EPSG:3857');
var zoom = map.getView().getZoom();
if (zoom < 12) {
typeahead.mapLocation = null;
} else {
typeahead.mapLocation = { lat: latlon[1], lon: latlon[0] };
}
});
The following figures show the results of biased typeahead:
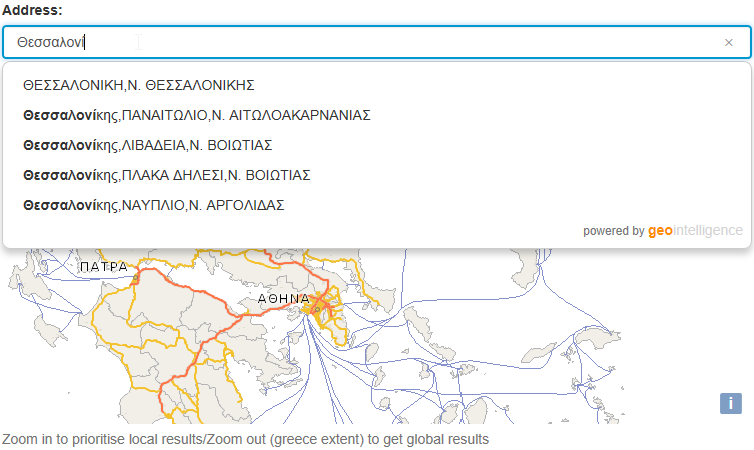
Results are not biased when zoomed out
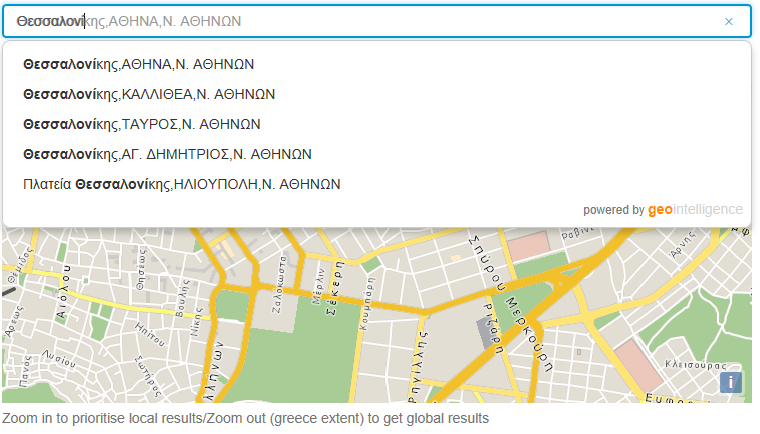
Results are biased using Athens as the anchor location
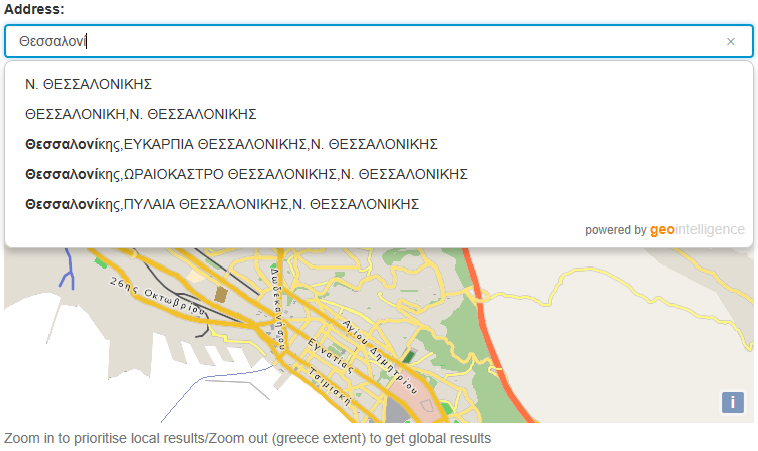
Results are biased using Thessaloniki as the anchor location