Search Address - Typeahead¶
A very common usage scenario in location-aware applications is enabling the user to find a location by specifying an address. This search operation is called geocoding and usually involves one ore more text fields where the user is required to enter the address in whole or in parts. No matter the implementation GIMS supports geocoding via a set of APIs.
This section aims to cover the most advanced geocoding case, which is letting the user specify the address in one input field providing at the same time typeahead feedback as shown in the following image.
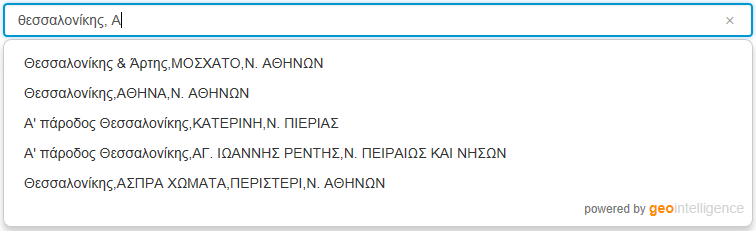
Typeahead while searching for an address
Typeahead¶
The sample is using GeoIntelligence’s mapperjs typeahead library. The library is based on Twitter’s typeahead.js library and internally uses the Mapper Server Maps API for address free text searching.
A lot of things happen under the hood when using mapperjs typeahead library:
- As the user types, the library uses the local search engine to get cached results that match (See more on typeahead.js for local search engine).
- When local results are not sufficient the library makes the necessary API calls to get results from the server.
- The library makes a smart call based on whether the user just entered text ignoring suggestions, used a suggestion or even selected a suggestion and appended additional information at the end. Using suggestions usually results to faster responses, so this can make a huge difference.
Note
Accessing GI Mapper Maps API to search for an address does not require using mapperjs typeahead library. GeoIntelligence has developed this library for the developers’ convenience as it handles most of the UX and API usage for them.
Setup Typeahead¶
Setting up typeahead is easy. The only things required is having access to GI Mapper Server, getting an API Key for your domain (see here for more info) and finally configure your web page appropriately.
Step 1: Include required scripts¶
The mapperjs typeahead library is available at https://maps.geointelligence.gr/lib/mapperjs/mapperjs.typeahead.bundle.min.js. If you own a GI Mapper Server instance then you can also find it on <path to your server>/lib/mapperjs/mapperjs.typeahead.bundle.min.js. That being said, we strongly recommend developers bundling the library in their web application.
<script src="lib/jquery/dist/jquery.min.js"></script>
<script src="lib/mapperjs/mapperjs.typeahead.bundle.min.js"></script>
Note
As aforementioned mapperjs typehead internally uses Twitter’s typeahead.js which has a dependency on jquery. Therefore jquery has to be included too
Step 2: Input field and Styling¶
Next developers have to use an input field with a certain id.
<input id="typeahead" type="text" placeholder="e.g. Λ Κηφισίας 97, Αθήνα"
class="typeahead"
autocomplete="off" />
Styling typeahead is specific to typeahead.js (see more on typeahead.js. The sample uses the following css:
.twitter-typeahead{
width:100%;
}
.typeahead {
background-color: #fff;
}
.typeahead:focus {
border: 2px solid #0097cf;
}
.tt-query {
-webkit-box-shadow: inset 0 1px 1px rgba(0, 0, 0, 0.075);
-moz-box-shadow: inset 0 1px 1px rgba(0, 0, 0, 0.075);
box-shadow: inset 0 1px 1px rgba(0, 0, 0, 0.075);
}
.tt-hint {
color: #999
}
.tt-menu {
width: 100%;
margin: 2px 0;
padding: 8px 0;
background-color: #fff;
border: 1px solid #ccc;
border: 1px solid rgba(0, 0, 0, 0.2);
-webkit-border-radius: 8px;
-moz-border-radius: 8px;
border-radius: 8px;
-webkit-box-shadow: 0 5px 10px rgba(0,0,0,.2);
-moz-box-shadow: 0 5px 10px rgba(0,0,0,.2);
box-shadow: 0 5px 10px rgba(0,0,0,.2);
}
.tt-suggestion {
padding: 3px 20px;
line-height: 24px;
}
.tt-suggestion:hover {
cursor: pointer;
color: #fff;
background-color: #0097cf;
}
.tt-suggestion.tt-cursor {
color: #fff;
background-color: #0097cf;
}
.tt-suggestion p {
margin: 0;
}
Step 3: Initialise Typeahead¶
The final step is initialising the typeahead control by using the appropriate options.
let typeahead = new gimapperjs.typeahead.MapperJSTypeahead({
// use a selector pointing to your input fields you want typeahead initialised
hostElementSelector: '#typeahead',
// pass the specified API Key
apiKey: apiKey,
// pass mapper server URI your API key has access to (e.g. https://maps.geointelligence.gr)
host: baseHost,
// Use true to get structured address components in typeahead results, false otherwise
fetchAddressComponents: false,
});
As shown here there is no map involved. The user just types an address and gets suggestions. We can do more, we could also show a map and on top of that let the location of the map bias the suggestions. This is the subject of the next section.